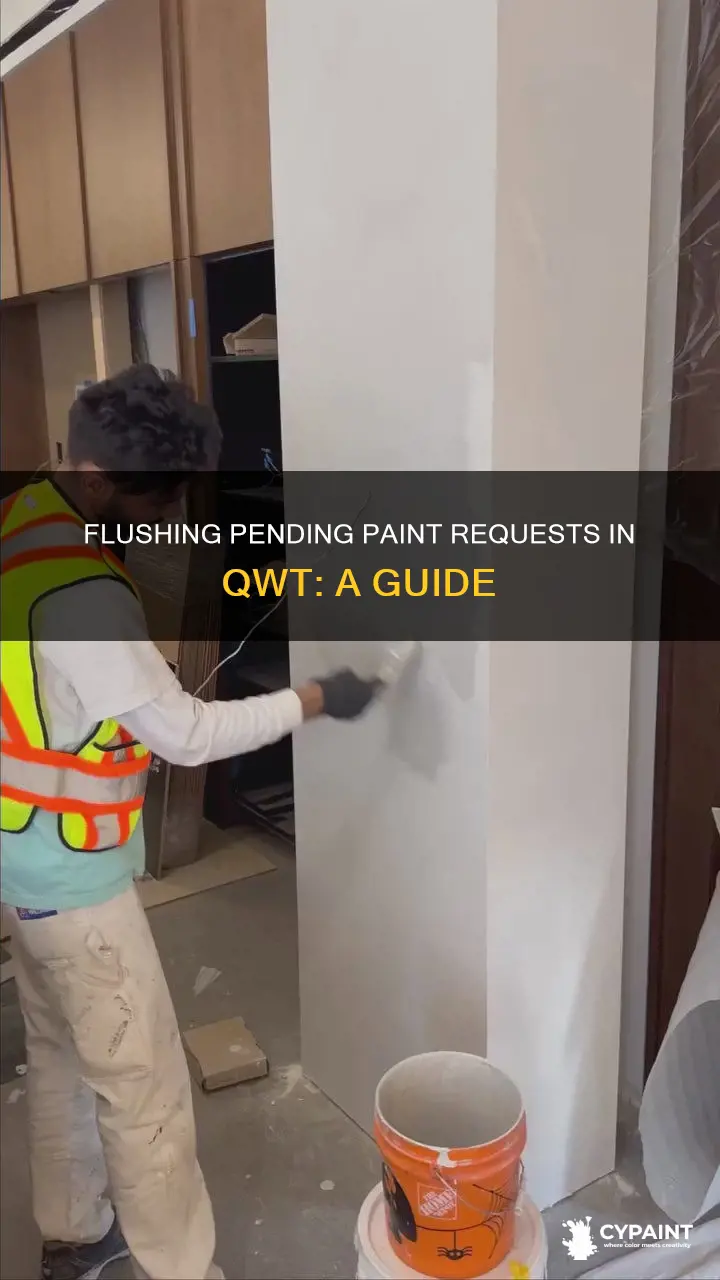
QwtPlotDirectPainter offers an API that allows users to paint subsets without erasing or repainting the entire plot canvas. This is especially useful when applications want to display samples while they are being collected. When there are too many samples, complete replots can be expensive to process in a collection cycle. While there is no clear consensus on how to flush all pending paint requests in Qwt, some workarounds include calling QWidget::update() after a set period and using the .
What You'll Learn
Using QPainter in Qt 4
QPainter is a rich framework that allows developers to perform a wide variety of graphical operations, such as gradients, composition modes, and vector graphics. It provides standard functions to draw points, lines, ellipses, arcs, Bézier curves, and other primitives.
The core functionality of QPainter is drawing, but the class also provides several functions that allow you to customise QPainter's settings and its rendering quality, and others that enable clipping. You can also control how different shapes are merged by specifying the painter's composition mode. The QPainter::CompositionMode is used to draw opaque objects onto a paint device. In this mode, each pixel in the source replaces the corresponding pixel in the destination.
To get the optimal rendering result, use the platform-independent QImage as the paint device. This will ensure that the result has an identical pixel representation on any platform. The QPainter class also provides a means of controlling rendering quality through its RenderHint enum and support for floating-point precision. All the functions for drawing primitives have a floating-point version. These are often used in combination with the RenderHint{QPainter::Antialiasing} render hint. The RenderHint enum specifies flags to QPainter that may or may not be respected by any given engine.
The common use of QPainter is inside a widget's paint event. Construct and customise the painter (e.g. set the pen or the brush), then draw. Remember to destroy the QPainter object after drawing. A painter is activated by the begin() function and the constructor that takes a QPaintDevice argument. The end() function and the destructor deactivate it.
Unveiling Artists: Finding Names Behind the Brush Strokes
You may want to see also
QwtPlotDirectPainter for painting subsets
QwtPlotDirectPainter is a tool that can be used to paint subsets without erasing or repainting the entire plot canvas. This is particularly useful when you want to display samples while they are being collected, as complete replots can be expensive to process when there are too many samples.
QwtPlotDirectPainter offers an API to paint subsets (for example, all additional points). It is important to note that in certain environments, it might be necessary to calculate a proper clip region before painting. For example, for Qt Embedded, only the clipped part of the backing store will be copied to a (possibly unaccelerated) frame buffer.
Incremental painting is only beneficial when no replot is triggered by another operation, such as changing scales, and nothing needs to be erased. When using QwtPlotDirectPainter, you can set plot()->canvas()->setAttribute(Qt::WA_PaintOutsidePaintEvent, true), which will result in faster painting if the paint engine of the canvas widget supports this feature.
To utilise QwtPlotDirectPainter for painting subsets, you can follow these steps:
- Assign a Clip Region and Enable Clipping: Depending on the environment, setting a proper clip region can significantly improve performance. For example, on Qt Embedded, only the clipped part of the backing store will be copied to a frame buffer device.
- Use QwtPlotDirectPainter to Paint the Subset: After setting up the clip region, you can use QwtPlotDirectPainter to paint your desired subset. This can be done without triggering a replot, which can be much faster.
- Optimise for Performance: If you are concerned about performance, you can consider subclassing the curve to cache itself when it is plotted. This way, when you replot, the curve can check what has changed, draw only the extra points, and then cache the new pixmap again.
- Handle Colour Changes: If you need to change the colour of data points, you can use QwtPlotDirectPainter to modify the colour without calling a replot. However, changing the colour back to its original state without replotting the entire QwtPlot or QwtPlotCurve can be challenging and may require deeper changes in the code.
- Clipping Data Points: When dealing with real-time data points, you might need to perform direct painting of new data points. By using QwtPlotDirectPainter, you can append the new data points to the curve data and then apply clipping if necessary on specific systems.
- Addressing Missing Plot Points: In some cases, you might encounter missing plot points during direct painting. This can be resolved by increasing the clipping region to the size of the canvas, ensuring that all data points are captured.
By following these steps and utilising the features of QwtPlotDirectPainter, you can efficiently paint subsets without the need for erasing or repainting the entire plot canvas.
Flipping Images in Medibang: A Step-by-Step Guide
You may want to see also
Drawing a set of points of a seriesItem
When it comes to drawing a set of points for a series item, the QwtPlotCurve class in Qwt provides a useful solution. A plot item in Qwt represents a series of points in the x-y plane, allowing for the creation of curves, markers, and other items that can be attached to axes.
To begin, it's important to understand the structure of a plot item. A plot item consists of a data object that contains the actual data points to be plotted. This data object can be configured to support different display styles, interpolation methods (such as spline), and symbols. By default, when a curve is created, it is set to draw black solid lines without any symbols.
The QwtPlotCurve class provides several methods to customize the appearance of the curve. One way to improve the performance of painting a large series of points is by using curve fitting. This involves executing a curve fitter on the curve points once and caching the result in a QwtSeriesData object. Additionally, you can specify the attributes for drawing the legend icon and assign symbols to the curve.
When working with a large number of points, it's important to consider the smoothness of the curve. While the QPainterPath class offers a "quadTo" function that produces relatively satisfying results for a lot of points, straight lines may not appear as aesthetically pleasing when dealing with a smaller set of points. In such cases, the "cubicTo" function might be considered, but it has limitations, such as only supporting three points and potentially introducing "braking points" at the connection of triplets.
Overall, the QwtPlotCurve class provides a flexible way to draw a set of points for a series item, allowing customization of curve appearance, handling of large data sets, and the ability to assign symbols to the curve.
Quick Tips to Fix a Poor Touch-Up Paint Job
You may want to see also
Modifying flags to avoid repainting
Firstly, obtain the current flags of the QWidget object using the QWidget::windowFlags() function. This allows you to retrieve the existing window flags, which control the widget's appearance, behaviour, and interaction with the desktop environment.
Next, modify the flags using bitwise operations (OR, AND, XOR) to add, remove, or toggle specific flags as needed. For example, you can use Qt::CustomizeWindowHint to disable default window decorations, Qt::FramelessWindowHint to hide the window frame, or Qt::WindowStaysOnTopHint to keep the widget always on top of other windows. You can also use the SetWindowLong function on Windows to modify window attributes, but be mindful of platform-specific behaviours and limitations.
After modifying the flags, call QWidget::setWindowFlags() with the modified flags to apply the changes. This ensures that the new flags are associated with the widget.
Finally, call QWidget::show() or QWidget::update() to force the widget to redraw itself with the new flags. This step is crucial to ensure that the changes are reflected visually.
It is important to carefully consider the impact of modifying window flags on other parts of your application. Test your code thoroughly to identify and address any unintended consequences, as modifying window flags may interfere with the behaviour of other Qt components, such as layouts or stylesheets. Additionally, ensure that the parent widget's layout and properties are compatible with the modified child widget's window flags.
Repairing Peeling Paint in Your Tub: A DIY Guide
You may want to see also
Using QPainter to draw text
The QPainter class is used for performing low-level painting on widgets and other paint devices. It provides a variety of functions for drawing lines, shapes, and text. To draw text using QPainter, you can use the drawText() function. This function is designed for drawing plain text without formatting. If you need to draw formatted or rich text, you should use QTextDocument::drawContents.
Here's an example of how to use QPainter to draw text:
QPainter painter(this);
Painter.setPen(Qt::blue);
Painter.setFont(QFont("Arial", 30));
Painter.drawText(rect(), Qt::AlignCenter, "Qt");
In this example, we first create a QPainter object and set the pen color to blue. Then we set the font to Arial with a size of 30. Finally, we use the drawText() function to draw the text "Qt" in the centre of the rect().
You can also use QPainter to draw text at a specific location. For example:
Painter.drawText(QPoint(10, 50), "Text");
This will draw the text "Text" at the coordinates (10, 50).
QPainter also supports drawing rich text with subscripts and superscripts using HTML-like tags. Here's an example:
QString equation = "Kmax=K2 . 3";
Painter.drawText(QRect(x, y, width, y+height), Qt::AlignLeft | Qt::AlignVCenter, equation);
In this example, the equation string contains subscript tags (sub) to indicate the subscripts in the text. The drawText() function is then used with a QRect to specify the bounding rectangle for the text, along with alignment options.
It's important to note that QPainter does not support text formatting directly within the drawText() function. If you need to draw formatted text, you should use QTextDocument::drawContents, as shown in the following example:
QPainter painter(this);
QTextDocument td;
Td.setHtml("Kmax=K2 · 3");
Td.drawContents(&painter);
In this example, the QTextDocument td is used to set the HTML content, which includes subscripts. The drawContents() function is then used to draw the formatted text using the QPainter object.
Finding Your 2003 Honda Civic's Paint Code
You may want to see also